This is my take on a “generic” tower defense game, as a school exercise. The game has only 1 tower type, which is a simple laser tower that shoots the closest enemy every x seconds. The tower does, on hit, deal a set amount of damage to the enemy hit. Towers can be placed on any valid tile. Valid tiles can be identified by bobbing up when the mouse hovers over them.
Time period | Engine & language(s) | Team size | GitHub link |
8-9 weeks | Unity, C# | 1 | here |
Process
Towers
Here is a flowchart of how the towers find the closest enemy:
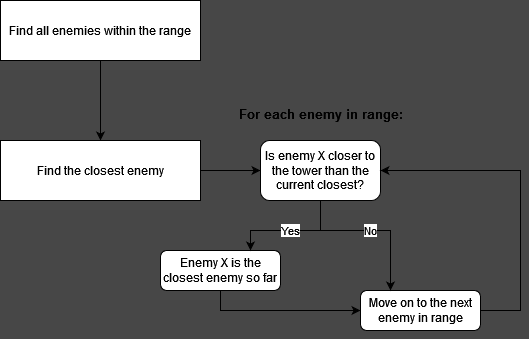
Here is how that looks in code:
View code
void FindClosestEnemy()
{
GameObject closest = null;
foreach(GameObject o in enemiesInRange)
{
if(closest != null)
{
if (Vector3.Distance
(o.transform.position, transform.position)
< Vector3.Distance
(closest.transform.position, transform.position))
{
closest = o;
}
}
else
{
closest = o;
}
}
target = closest;
}
Shooting
For the shooting of the enemies, the towers use the following code, among other parts:
View code
void ShootEnemyLaser(GameObject t)
{
Vector3 raypos = new Vector3
(transform.position.x, transform.position.y + 1.0f,
transform.position.z);
Vector3 targetpos = new Vector3
(t.transform.position.x, t.transform.position.y + 0.25f,
t.transform.position.z);
Vector3 dir = -(raypos - targetpos);
Ray ray = new Ray(raypos, dir);
RaycastHit hitData;
Physics.Raycast(ray, out hitData);
Vector3[] linePositions = {raypos, targetpos};
StartCoroutine(CreateLaserLines(linePositions));
GameObject hitObj = null;
hitObj = hitData.transform.gameObject;
if(hitObj != null)
{
if (hitObj.tag == "enemy")
{
try
{
EnemyController enemy = hitObj.
GetComponent<EnemyController>();
if (enemy.currentHealth > 0)
{
enemy.TakeDamage(damage);
}
}
catch
{
return;
}
}
}
}
The function does roughly the following:
- Create a Ray;
- Raycast using the Ray, and store the hitData;
- Start a coroutine that handles the rendering of the laser;
- Check if the raycast hit an object, and if it is an enemy;
- If the raycast hit an enemy, damage the enemy using a function on the enemy controller.
You can find the GitHub repository here.